Clean Architecture
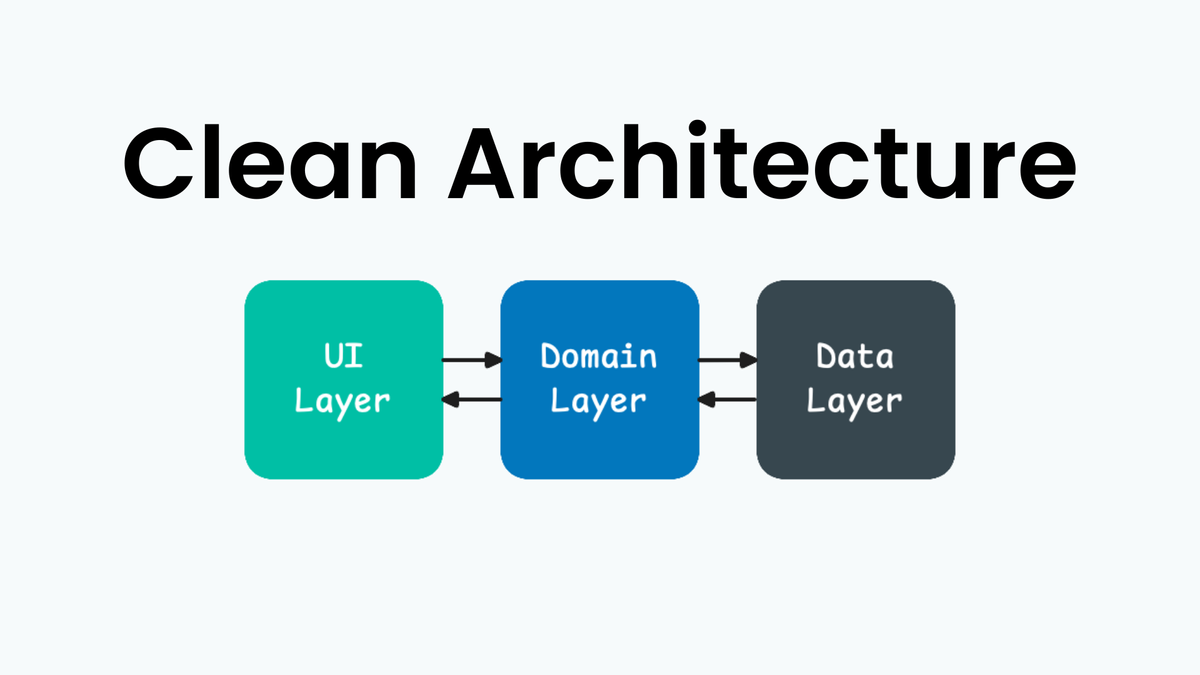
Now, I am beginning series of related articles needed to create mid to big applications in a scalable, maintainable and testable way.
Table of contents:
- What is Clean Architecture?
- Presentation Layer
- Domain Layer
- Data Layer
- Summary
- What is next?
- References
What is Clean Architecture?
There are many articles out there that explain Clean Architecture, but still, everyday new articles are out on the subject. When speech goes about Clean Architecture, we all remember the diagram below.
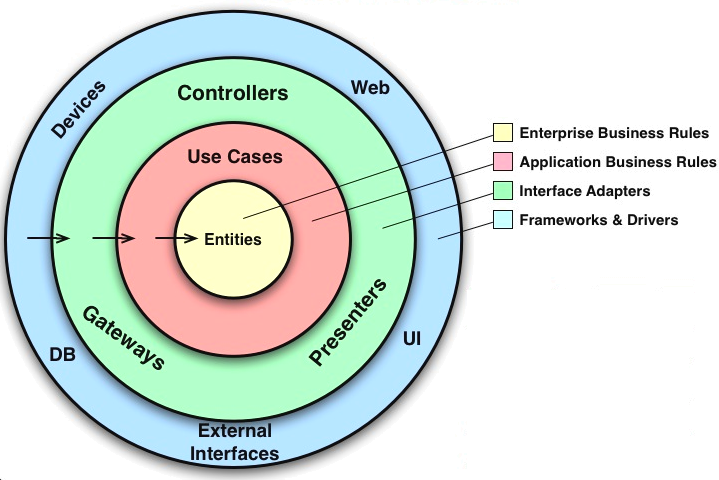
Some engineers think whole Clean Architecture's idea is all this and try to learn the picture by heart and retell the same picture when asked to explain, without understanding it. However, there is deep idea behind Clean Architecture and this photo is a single implementation of it.
Clean Architecture is a software design philosophy that emphasizes organizing the system in a way that separates concerns, promotes scalability, and makes systems easier to test and maintain. It was introduced by Robert C. Martin (Uncle Bob).
Let's talk about the highlighted principles above with understandable terms. We have to build a car. We don't build all the details of a car in one place rather a car consists of many components built separately. For example, car has wheels, engine, body, seats, radio, software which are all created in separate factories - separating concerns.
Now, the question is why we need to separate concerns? Let's say we want to produce more cars. We see that we have all the details but lacking engines to build more cars. What we do is to enlarge the engine factory to make it capable of producing more engines. We achieve this by breaking the car into components. We see that our factories are scalable individually now.
When we separate details, we can always improve the quality by testing them in place. Let's say in a factory that creates radio for our car, the focus of the factory is on only one thing - radio. They try to perfect their product, they know what and how to test. Moreover, if let's say there's defect in the product they found out after production, the factory can easily provide maintenance for the particular component.
So, obeying Clean Architecture principles, in our mobile application world, we mainly divide the application into presentation, domain and data layers.
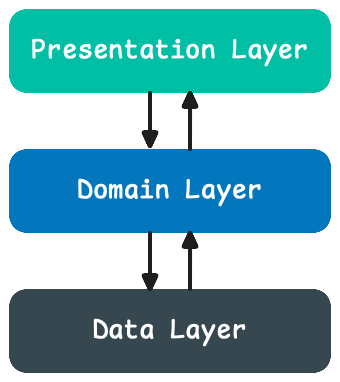
Presentation Layer
The role of this layer is to display application data on the screen. This layer is responsible to react to user interactions, pass the interaction to domain layer or reflect the changes coming from data layer. It consists of two components: UI elements (widgets/dialogs/bottom-sheets) and UI state holders (Bloc/ViewModel).
UI elements are responsible for displaying the data on screen through widgets, dialogs, bottom-sheets and more, it is an interface to get user interactions as well and pass the interactions to domain layer. They should not contain business logic, as this resides in the domain layer. UI elements can be tested through golden tests, just FYI.
UI state holders stay behind UI elements and keep the state, handle UI logic, mediate between the view and the underlying application logic. Some examples are ViewModels, Presenters, Controllers or Bloc for Flutter. By separating UI elements and UI state holders, the system gains separate testability as well.
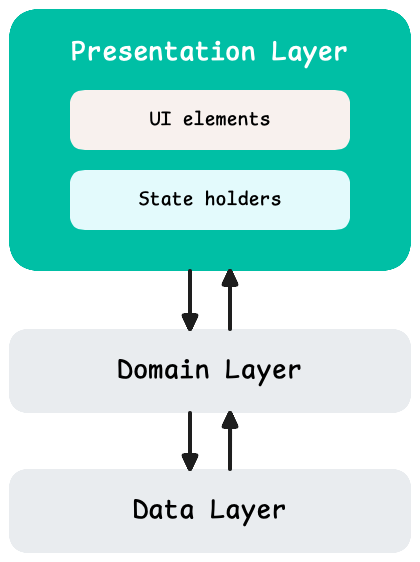