Flutter Custom Painter: Creating Stunning UI with Custom Painting
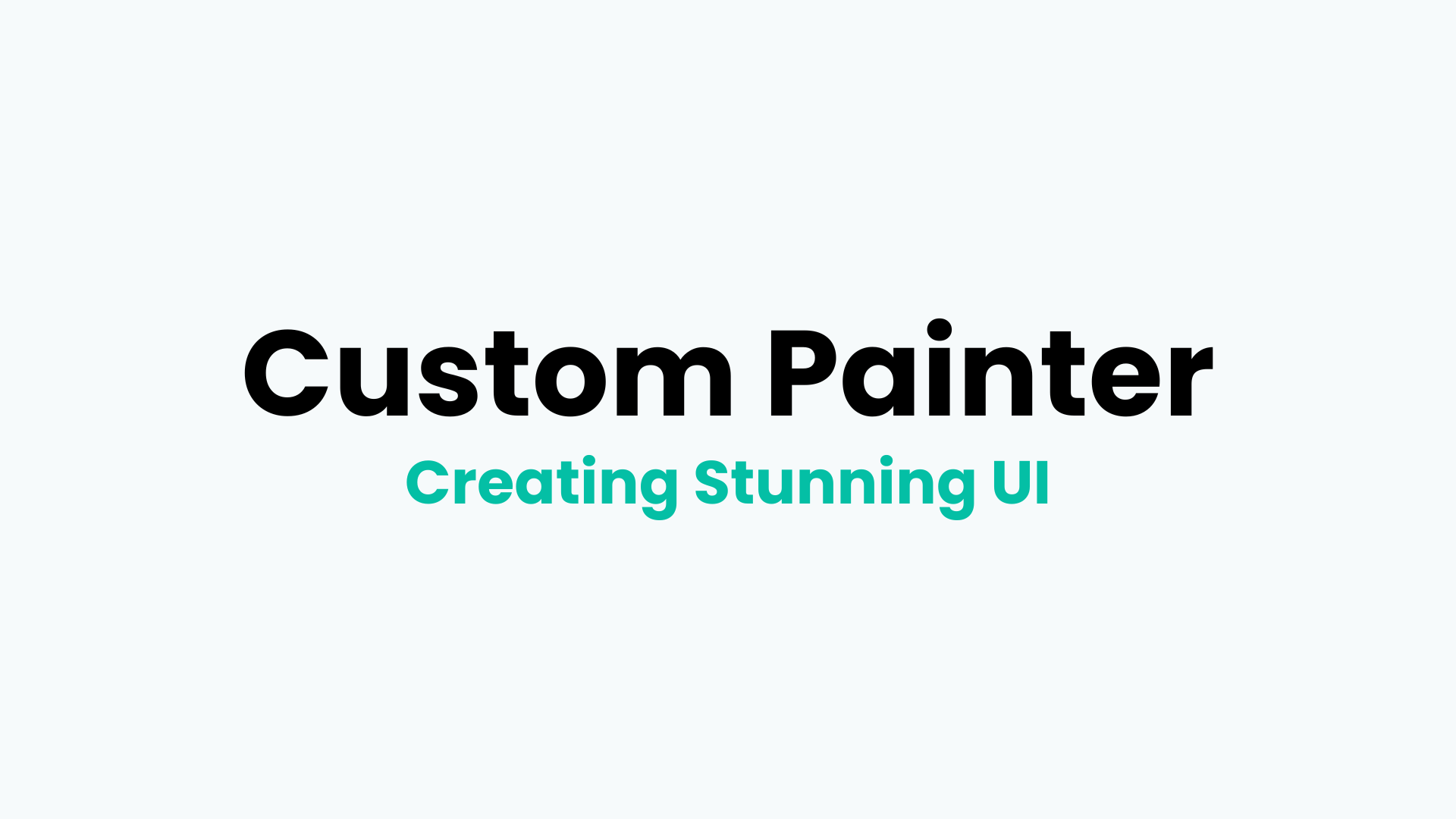
Flutter provides a rich set of built-in widgets, but sometimes you need to create custom UI elements that are not available by default. This is where CustomPainter comes in. It allows you to draw shapes, paths, and complex designs directly on the screen. In this article, we will explore how CustomPainter works and how to use it to create custom UI elements in Flutter.
Table of contents:
- What is CustomPainter?
- When to use CustomPainter?
- Steps to create CustomPainter
- Basic Example: Drawing a Circle
- Advanced Example: Drawing a Wave
- Animating CustomPainter
- Conclusion
- References
What is CustomPainter?
CustomPainter
is a Flutter class that enables you to draw custom graphics using the Canvas API. It allows you to create shapes, lines, text, and even animations by overriding the paint
method.
When to use CustomPainter?
- Draw custom shapes (e.g., waves, arcs, polygons).
- Create complex UI elements beyond Flutter’s built-in widgets.
- Optimize performance by reducing widget rebuilds.
- Implement graphs, and interactive drawings.
Steps to create CustomPainter
To use CustomPainter, you need to:
- Create a Custom Painter class by extending
CustomPainter
. - Override the
paint
method to draw usingCanvas
. - Override the
shouldRepaint
method to define when the painter should repaint. - Use
CustomPaint
widget to display the drawing in your Flutter app.
Basic Example: Drawing a Circle
Let’s start with a simple example: drawing a red circle using CustomPainter
.
Step 1: Create the Custom Painter Class
import 'package:flutter/material.dart';
class CirclePainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
final Paint paint = Paint()
..color = Colors.red
..style = PaintingStyle.fill;
final Offset center = Offset(size.width / 2, size.height / 2);
final double radius = size.width / 3;
canvas.drawCircle(center, radius, paint);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
return false;
}
}
Step 2: Use CustomPaint to Display the Circle
import 'package:flutter/material.dart';
import 'circle_painter.dart'; // Import the custom painter file
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Custom Painter Example")),
body: Center(
child: CustomPaint(
size: Size(200, 200), // Define the canvas size
painter: CirclePainter(),
),
),
),
);
}
}
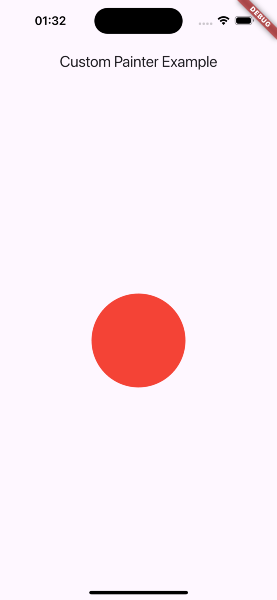
Advanced Example: Drawing a Wave
Let’s create a more complex shape — a wave pattern.
Step 1: Create the WavePainter Class
import 'package:flutter/material.dart';
class WavePainter extends CustomPainter {
@override
void paint(Canvas canvas, Size size) {
Paint paint = Paint()
..color = Colors.blue
..style = PaintingStyle.fill;
Path path = Path();
path.moveTo(0, size.height * 0.7);
path.quadraticBezierTo(
size.width * 0.25, size.height * 0.5,
size.width * 0.5, size.height * 0.7
);
path.quadraticBezierTo(
size.width * 0.75, size.height * 0.9,
size.width, size.height * 0.7
);
path.lineTo(size.width, size.height);
path.lineTo(0, size.height);
path.close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) {
return false;
}
}
import 'package:flutter/material.dart';
import 'wave_painter.dart'; // Import the wave painter
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Stack(
children: [
CustomPaint(
size: Size(double.infinity, 300),
painter: WavePainter(),
),
Center(
child: Text(
"Custom Wave",
style: TextStyle(fontSize: 24, color: Colors.white),
),
),
],
),
),
);
}
}
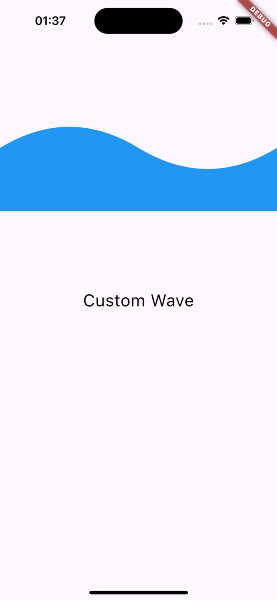
Animating CustomPainter
To animate a CustomPainter
, use CustomPaint
with AnimatedBuilder
. Let’s animate the wave movement.
Step 1: Modify the WavePainter Class
class AnimatedWavePainter extends CustomPainter {
AnimatedWavePainter(this.waveOffset);
final double waveOffset;
@override
void paint(Canvas canvas, Size size) {
Paint paint = Paint()
..color = Colors.blue
..style = PaintingStyle.fill;
Path path = Path();
path.moveTo(0, size.height * 0.7);
path.quadraticBezierTo(
size.width * 0.25, size.height * (0.5 + waveOffset),
size.width * 0.5, size.height * (0.7 - waveOffset)
);
path.quadraticBezierTo(
size.width * 0.75, size.height * (0.9 + waveOffset),
size.width, size.height * (0.7 - waveOffset)
);
path.lineTo(size.width, size.height);
path.lineTo(0, size.height);
path.close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(covariant AnimatedWavePainter oldDelegate) {
return oldDelegate.waveOffset != waveOffset;
}
}
class AnimatedWave extends StatefulWidget {
@override
_AnimatedWaveState createState() => _AnimatedWaveState();
}
class _AnimatedWaveState extends State<AnimatedWave> with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<double> _animation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: Duration(seconds: 2),
vsync: this
)..repeat(reverse: true);
_animation = Tween<double>(begin: -0.1, end: 0.1).animate(_controller);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: _animation,
builder: (context, child) {
return CustomPaint(
size: Size(double.infinity, 300),
painter: AnimatedWavePainter(_animation.value),
);
},
);
}
}
Step 3: Use the Animated Wave in Your App
void main() {
runApp(
MaterialApp(
home: Scaffold(
body: AnimatedWave(),
),
),
);
}
Conclusion
CustomPainter
lets you draw custom UI elements using the Canvas API.- You can draw shapes, paths, gradients, and animations for unique designs.
- By combining
CustomPaint
with animations, you can create dynamic, interactive UIs. - Use
shouldRepaint
wisely to optimize performance.
CustomPainter
is a powerful tool that unlocks endless creative possibilities in Flutter. Whether you're designing unique UI elements, data visualizations, or animated effects, CustomPainter
is the way to go!